Application hook
How to configure the application hook, which allows your product to create applications based on previously generated quotes
Overview
Once the end-customer accepts a quote, an application can be created based on that quote. The application step is typically used to gather and save further information, which is not relevant for calculating the premium, to the policy.
This is implemented on Root by means of the application hook, which follows the following sequential steps:
- Additional policy information, from user input and/or existing system data, is combined and passed to the application API endpoint from your system or the Root dashboard.
- This data is then fed to a validation function you define, called
validateApplicationRequest()
. - Once validated, this data is fed to the
getApplication()
function where the data can be manipulated. getApplication()
returns anApplication
object back through the API synchronously as the response to the initial API request. The application is also saved on Root and is later used to issue the policy.
Building the application hook is analogous to building the quote hook. You are configuring the application endpoint for the product you are building, using the product module code.
Linking an application to a policyholder
Creating an application requires not only a quote (QuotePackage
) to exist, but also a policyholder. The application request needs to reference the policyholder's policyholder_id
.
The process by which policyholders are created is standard across product modules, and relies on a separate policyholder endpoint, which does not need to be configured in the product module code.
Typically, a new policyholder will be created before creating the application. However, the application can also be linked to an existing policyholder created previously.
Specifying application parameters
Since an application is created based on an existing quote, the rating factors used to calculate the premium will most likely already have been used and saved at the quote step. Therefore, the input parameters for the application step typically represent additional information that does not affect the premium or quote.
This can be a combination of data captured from the user and extra information that's already known on your system. For example, for some products it is useful to save information about the relevant distribution channel or the sales agent at the application step.
Below is an example of an application payload for our Dinosure life insurance product.
{
"quote_package_id": "123aaf98-093c-4103-989f-5c4286e4f417",
"policyholder_id": "5c64f040-dcd9-443f-b359-2b1a56e8c1c8",
// Extra info that does not affect the premium
"requires_early_warning_radio": true,
"heli_landing_pad_near_home": false,
// Did someone refer this person, perhaps they need commission
"referral": true,
"referrer_member_id": "dino_1337",
}
The quote_package_id
and policyholder_id
are standard fields for all product modules. These values can be obtained from the quote package and policyholder objects returned by the quote endpoint and the policyholder endpoint.
The other fields are product-specific and you can determine which fields to include based on the product specification. In the example provided, the additional application fields are not required to calculate the premium, but are saved to the policy for use in the event of a claim.
The JSON data in the application request payload is parsed and injected as the data
parameter in the validateApplicationRequest()
function for validation.
Dashboard dependency
If the Root dashboard will be used to issue policies for your product, the application request payload structure needs to match the application schema used to capture information through the user interface.
Validating the application parameters
Validating the application request data is an analogous to validating the quote request data. The name of the function is validateApplicationRequest()
and it also relies on the Joi validation library. Below is a skeleton example.
validateApplicationRequest()
receives not only the input data
as an argument, but also the policyholder
and quotePackage
objects, as specified in the application request payload. These objects can be used to perform cross-validation between the application input data, and the quote and policyholder objects already saved to the platform. For example, it may be useful to validate the policyholder's ID number or date of birth against her age received at the quote stage.
/**
* Validates the application request data.
* @param {Record<string, any>} data The data received in the body of the
* <a href='https://docs.rootplatform.com/reference/getting-a-quote-2' target='_blank'>Create an application</a> request
* (without the `policyholder_id`, `quote_package_id`, and `billing_day` properties).
* @param {PlatformPolicyholder} policyholder The policyholder that will be linked to the application
* @param {PlatformQuotePackage} quote_package The quote package from which the application is created
* @return {{error: any; result: any}} The <a href='https://joi.dev/api/?v=12.1.0#validatevalue-schema-options-callback' target='_blank'>validation result</a>.
* If there are no errors, `result.value` property will contain the validated data, which is passed to `getApplication`.
* @see {@link https://docs.rootplatform.com/docs/application-hook Application hook}
*/
const validateApplicationRequest = (data, policyholder, quote_package) => {
// Custom validation can be specified in the function body
const validationResult = Joi.validate(
data,
Joi.object().keys({
// The Joi validation schema can be completed here
}).required()
);
return validationResult;
}
If no errors are thrown, the validated input data returned by this function is passed to the getApplication()
function by the platform.
Creating an application
The getApplication()
function is analogous to the getQuote()
function. Here, the input data can be manipulated and organised (if required) before it is saved to the application module.
One key difference is that getApplication()
accepts three arguments instead of one: the validated application request input data, a quote package object and a policyholder object. It returns an application object. A skeleton example is provided below.
/**
* Generates an application from the application request data, policyholder and quote package.
* @param {Record<string, any>} data The validated data, returned by `validateApplicationRequest` as `result.value`.
* @param {PlatformPolicyholder} policyholder The policyholder that will be linked to the application
* @param {PlatformQuotePackage} quote_package The quote package from which the application is created
* @return {Application} The application that will be returned by the
* <a href='https://docs.rootplatform.com/reference/create-an-application' target='_blank'>Create an application</a> endpoint.
* @see {@link https://docs.rootplatform.com/docs/application-hook Application hook}
*/
const getApplication = (data, policyholder, quote_package) => {
const application = new Application({
// The top-level fields are standard across all product modules
package_name: quote_package.package_name,
sum_assured: quote_package.sum_assured,
base_premium: quote_package.base_premium,
monthly_premium: quote_package.suggested_premium,
input_data: {...data},
module: {
// The module object is used to store product-specific fields
...quote_package.module,
...data,
},
});
return application;
}
The top-level fields under the Application
object, such as sum_assured
and monthly_premium
are standard for all product modules. See the API reference for the Application
object for details on what these fields represent.
Custom, product-specific information is saved in the module
object for later reference. Typically, rating factors received at the quote stage (saved in the quote package's module object) will be carried over and included in the application module.
Converting module properties to currencies on dashboard
If a property in the module data has one of the following keywords, it'll be converted to a currency value on the dashboard:
premium
,amount
,income
,assured
,value
andfund
.For example,
basic_income_per_month: 1990000
will render as$ 19,900.00
. The currency symbol is determined by the billing settings.
The application object returned by this function is saved to the platform with its own application_id
, and then returned over the API. An application represents a complete "draft" policy. That is to say, all the required policy information has been captured and saved. The next step is to issue the policy using the relevant application_id
.
Dashboard application workflow
If your product's policies will be issued from the Root dashboard, you will need to configure the application schema (workflow) for this product module. This involves specifying the input components (form elements) that will be displayed to the user on the dashboard's application screen, and to which key in the application request payload each input component corresponds.
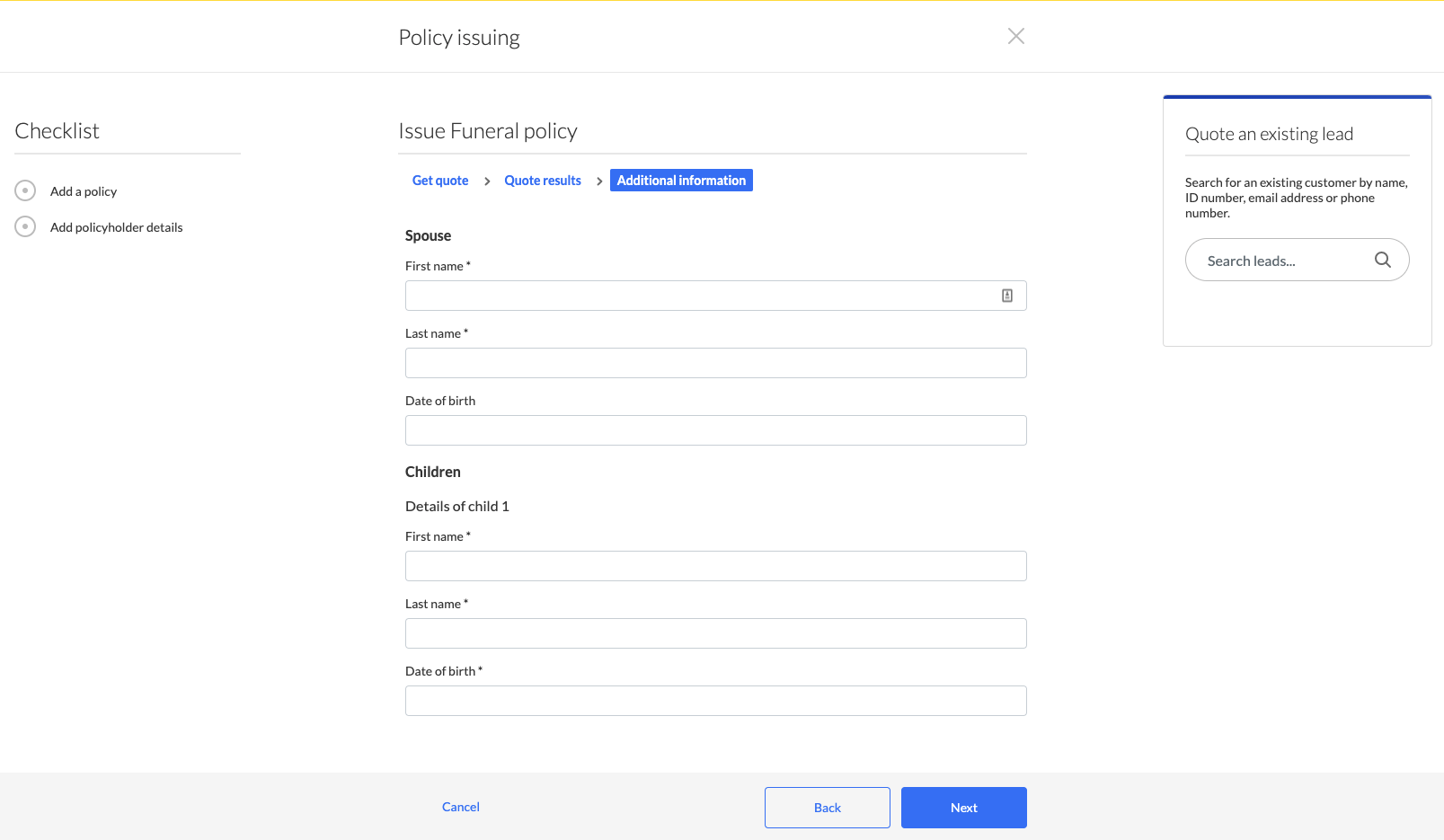
The Root dashboard application screen, as defined in the application schema for the Root Funeral product.
This aspect is independent of the application endpoint, and is not needed for API-only applications. However, if your product does rely on the Root dashboard for policy issuing. it's critical to ensure that the schema matches the expected application parameters.
Please see the schema guide for more details on configuring workflows for the dashboard.
Updated 5 months ago