Reactivation hook
How to enable and specify custom logic for reactivating policies
Overview
Reactivating a policy means changing the status of an inactive policy (for example a cancelled or lapsed policy) to active. This means the end-customer will once again be able to claim under the policy, and payments will once again be collected from the customer.
Reactivation can be enabled or disabled in the general product settings. If reactivation is enabled, the reactivation options need to be defined in the product module code in a function called getReactivationOptions()
.
Policies are reactivated using the reactivation API endpoint. Defining the reactivation options means configuring how this endpoint will function for your product. This also determines which reactivation options will be displayed on the Root dashboard.
Defining reactivation options
Below is an example of the getReactivationOptions()
function.
const getReactivationOptions = (policy) => {
const settlementAmount = policy.balance < 0 ? -policy.balance : 0;
return [
new ReactivationOption({
type: 'reinstatement',
settlementAmount,
description: 'For a policy to be reinstated, all arrear premiums will immediately be due.',
minimumBalanceRequired: true,
}),
new ReactivationOption({
type: 'recommencement',
description: 'For a policy to be recommenced, all arrear premiums will be deducted from the first claim income.',
minimumBalanceRequired: false,
}),
];
}
This function accepts a policy object as an argument, and returns an array of reactivation options. This means unique reactivation options can be made available based on the specifics of the policy (e.g. certain benefits, or how long the policy has been on the books).
The relevant reactivation option must be selected by the dashboard user (if reactivating the policy through the front-end), or passed to the reactivation endpoint (if reactivating through the API).
The names and descriptions of the different reactivation options will appear on the dashboard reactivation modal.
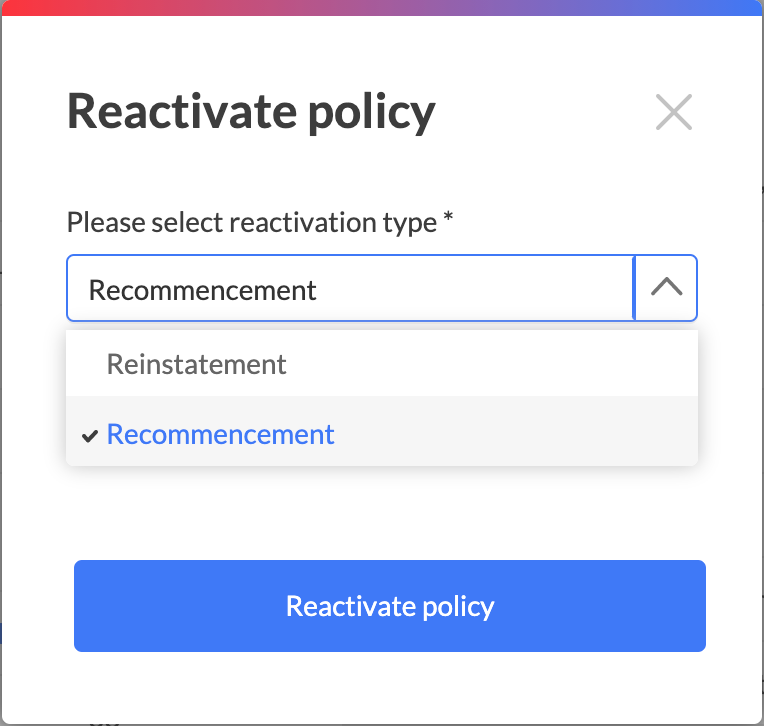
Dashboard reactivation modal showing reactivation options as defined in the getReactivations()
function in the product module code.
The type
field of the reactivations option does not, in itself, determine how the reactivation will be handled by the platform. There are no pre-defined reactivation types. You can choose the names of the reactivation options based on the product requirements.
If they are not constrained or defined further, all reactivation options will perform the same action by default, namely changing the status of the policy to "active".
Constraining reactivation based on the policy balance (outstanding arrears)
One way to constrain a reactivation option is by setting the minimumBalanceRequired
field to true
. This limits policies that can be reactivated to those where the arrears balance falls below a specified amount.
The settlement amount that has to be paid by the customer to enable reactivation is set using the settlementAmount
field. For example, setting settlementAmount
equal to policy.balance
means the full arrears balance needs to be settled by the customer before the policy can be reactivated.
Reactivation option object reference
Field | Description |
---|---|
type | String. The name of the reactivation option as it will appear on the dashboard, and which must be passed to the reactivation endpoint. |
description | String. The description of the reactivation option as it will appear on the dashboard. |
minimumBalanceRequired | Boolean. Whether reactivation is only allowed if the (negative) balance on the policy falls below a minimum absolute amount. If set to false, all policies can be reactivated irrespective of the arrears amount outstanding. |
settlementAmount optional | Integer. Required if minimumBalanceRequired is true . The amount, in cents, that must be settled by the customer to allow the policy to be reactivated. Must be larger than zero. |
Configuring reactivation behaviour
It may be required to limit reactivation to certain conditions, or to define specific behaviour for different reactivation options. In these cases, the lifecycle hooks beforePolicyReactivated()
and afterPolicyReactivated()
can be used. See the lifecycle hooks guide for more details.
Below is an example of a beforePolicyReactivated()
hook. It takes a policy object and a reactivation option object as arguments. The reactivation option passed to this hook will be the one selected by the dashboard user, or the one specified in the reactivation request payload.
/**
* Executed before a policy is reactivated.
* Can be used to prevent reactivation if certain conditions are not met.
* @param {object} params
* @param {PlatformPolicy} params.policy The policy to be reactivated
* @param {ReactivationOption} params.reactivationOption The reactivation option selected
* @return {ProductModuleAction[] | void} The actions to be queued by the platform
*/
const beforePolicyReactivated = ({ policy, reactivationOption, policyholder }) => {
// Check policy status is lapsed or cancelled
const isPolicyLapsedOrCancelled = ['lapsed', 'cancelled'].includes(policy.status);
if (!isPolicyLapsedOrCancelled) {
throw new Error(
`Policy with status ${policy.status} cannot be reactivated. Policy status must be one of ['lapsed', 'cancelled'].`,
);
}
// Check policy was active within the last six months
const sixMonthsFromUpdated = moment(policy.status_updated_at).add(6, "months")
if (isPolicyLapsedOrCancelled && moment().isAfter(sixMonthsFromUpdated)) {
throw new Error(`Policy can only be reactivated within 6 months of lapse or cancellation. Latest reactivation date was ${sixMonthsFromUpdated.format("YYYY-MM-DD")}`);
}
};
In this example, the function restricts reactivation 1) to policies that have a status of lapsed or cancelled, and 2) to policies that lapsed or were cancelled in the past six months. If these conditions are not met, an error is thrown, preventing reactivation from being completed.
This can also be defined in getReactivationOptions()
in order to not display the option in the dashboard menu in the first place. This will improve the dashboard reactivation workflow, with the trade-off that some reactivation options will be completely unavailable for some policies, which may not be transparent if policies are reactivated via the API only.
Reactivation and consecutive missed payments
Before enabling reactivation for your product module, you need to consider how the reactivation logic will interact with your product module’s lapse rules. For example, the “consecutive missed payments” lapse rule will not automatically take into account whether a policy has been reactivated.
Let’s assume the maximum number of consecutive missed payments according to the product module settings is 4. After the fourth missed payment, the policy is lapsed by the platform. If the policy is now reactivated, it will shortly lapse again, since the last four payments recorded on the policy remain failed.
If you want to prevent this behaviour you need to ensure that, by the time the policy is reactivated, the consecutive missed payments rule no longer applies. These are some examples of how you can achieve this:
- Use the
beforePolicyReactivated
lifecycle hook to prevent reactivation of policies in arrears. This will ensure that policies cannot be reactivated without past missed payments first being collected successfully. See the “Constraining reactivation based on the policy balance” section above. - Manually create an ad hoc payment prior to reactivation. Before reactivation, allocate an ad hoc payment to settle the policy arrears on the Root management dashboard.
- Use the
beforePolicyReactivated
lifecycle hook to create a successful payment. If the intention behind reactivation is always to forgive policy arrears, you can use this hook to automatically create a successful payment equal to the outstanding balance every time a policy is reactivated. The platform will not attempt to collect payments that already have a status ofsuccessful
. - Use the
beforePolicyReactivated
lifecycle hook to create a pending payment. You can use this hook to automatically create a pending payment equal to the outstanding balance plus the monthly premium amount. Platform will attempt collection of the pending payment before the policy is lapsed. However, if this payment fails, the policy will lapse again due to the consecutive missed payment rule being met.
Updated 11 months ago